Node.js의 프로세스 매니저 PM2
Node.js는 싱글스레드로 Node.js 애플리캐이션은 단일 CPU 코어에서 실행된다.
따라서 CPU의 멀티코어시스템을 사용할 수 없다. 이 문제를 해결하기 위해 클러스터(Cluster) 모듈을 통해 단일 프로세스를 멀티 프로세스(Worker)로 늘릴 수 있는 방법을 제공한다. 즉, 클러스터 모듈을 사용해서 마스터 프로세스에서 CPU 코어 수 만큼 워커 프로세스를 생성해 모든 코어를 사용하게 개발하면된다.
PM2의 등장 배경
애플리케이션을 실행하면 처음에는 마스터 프로세스만 생성된다. 이때 CPU 개수만큼 워커 프로세스를 생성하고 마스터 프로세스와 워커 프로세스가 각각 수행해야 할 일들을 정리해서 구현하면 된다. 예를 들어 워커 프로세스가 생성됐을 때 온라인 이벤트가 마스터 프로세스로 전달되면 어떻게 처리할지, 워커 프로세스가 메모리 제한선에 도달하거나 예상치 못한 오류로 종료되면서 종료(exit) 이벤트를 전달할 땐 어떻게 처리할지, 그리고 애플리케이션의 변경을 반영하기 위해 재시작해야 할 때 어떤 식으로 재시작을 처리할 지 등등 고민할 게 많다. 이런 것들은 직접 개발하기에는 번거로운데 PM2라는 Node.js 프로세스 매니저를 통해 처리할 수 있다.
ecosystem.config.js (자세하게 추가 공부)
module.exports = {
apps: [
{
name: 'dybsoft-front', // pm2 name
script: 'yarn', // // 앱 실행 스크립트
args: 'start',
instances: 4, // 클러스터 모드 사용 시 생성할 인스턴스 수
exec_mode: 'cluster', // fork, cluster 모드 중 선택
merge_logs: true, // 클러스터 모드 사용 시 각 클러스터에서 생성되는 로그를 한 파일로 합쳐준다.
autorestart: true, // 프로세스 실패 시 자동으로 재시작할지 선택
watch: false, // 파일이 변경되었을 때 재시작 할지 선택
// max_memory_restart: "512M", // 프로그램의 메모리 크기가 일정 크기 이상이 되면 재시작한다.
env: {
//환경변수 모든 배포환경에서 공통으로 사용
PORT: 80,
NODE_ENV: 'development',
},
env_production: {
// 운영 환경설정 (--env production 옵션으로 지정할 수 있다.)
NODE_ENV: 'production',
}
},
]
};
Nginx, pm2 와 관계, 배포설정
1. nginx를 앞에 두고 build 파일을 연결시킬 때 nginx.conf 파일은 아래와 같이 설정
* location 부분 차이 잘 보기
## http -> https로 redirect
server {
listen 80;
server_name dybsoft.com;
return 301 https://www.dybsoft.com$request_uri;
}
server {
listen 443 ssl;
server_name dybsoft.com;
ssl_certificate /app/ssl/dybsoft-ssl.crt;
ssl_certificate_key /app/ssl/dybsoft-ssl.key;
# react로 들어올 때
location / {
root /app/build;
index index.html;
try_files $uri $uri/ /index.html;
}
# apiserver proxy 설정
location /api {
proxy_pass http://192.168.0.105:7080;
proxy_http_version 1.1;
proxy_cache_bypass $http_upgrade;
}
# error_page 500 502 503 504 /50x.html;
# location = /50x.html {
# root /usr/share/nginx/html;
# }
}
2. nginx는 reverse proxy 역할만 하게 설정할 경우
proxy_pass에 react, apiserver 별도로 두고, react는 pm2로 node 프로세스 관리
* 여기서 하나의 인스턴스에 api 서버와 react를 같이 돌리기 때문에 192.168.0.105로 private IP 동일한 것
## http -> https로 redirect
server {
listen 80;
server_name dybsoft.com;
return 301 https://www.dybsoft.com$request_uri;
}
server {
listen 443 ssl;
server_name dybsoft.com;
ssl_certificate /app/ssl/dybsoft-ssl.crt;
ssl_certificate_key /app/ssl/dybsoft-ssl.key;
# react로 들어올 때
location / {
proxy_pass http://192.168.0.105:3000;
proxy_http_version 1.1;
proxy_cache_bypass $http_upgrade;
}
# apiserver proxy 설정
location /api {
proxy_pass http://192.168.0.105:7080;
proxy_http_version 1.1;
proxy_cache_bypass $http_upgrade;
}
# error_page 500 502 503 504 /50x.html;
# location = /50x.html {
# root /usr/share/nginx/html;
# }
}
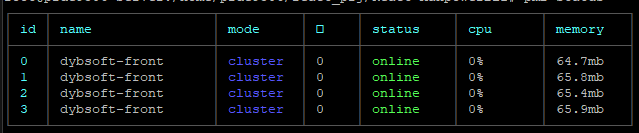
https://engineering.linecorp.com/ko/blog/pm2-nodejs
PM2를 활용한 Node.js 무중단 서비스하기
이 글은 마이크로소프트웨어 393호에 기고된 글입니다. 자바스크립트는 가장 널리 사용되는 클라이언트 측 프로그래밍 언어이자 프론트엔드 웹 개발 언어 중 하나입니다. 그리고 Node.js는 Chrome의
engineering.linecorp.com
nginx에서 node로 로드밸런싱
If you are using Nginx as a reverse proxy in front of your React front-end app deployed with PM2, Nginx will handle the incoming requests and distribute them evenly across the instances managed by PM2. This is achieved through Nginx's load balancing capabilities.
When multiple instances of your React app are running under PM2, Nginx acts as the entry point and distributes the incoming requests across these instances based on the configured load balancing algorithm. By default, Nginx uses a round-robin algorithm, which evenly distributes requests in a sequential manner to each instance.
Here's how the flow typically works:
- User sends a request to your application, targeting a specific URL.
- The request first reaches Nginx, which acts as a reverse proxy.
- Nginx examines the request and determines which instance(s) of your React app should handle the request based on the configured load balancing algorithm.
- Nginx forwards the request to the chosen instance(s) of your React app.
- The selected instance(s) receives the request and processes it, generating a response.
- The response is sent back through Nginx to the user who initiated the request.
By using Nginx as a reverse proxy in front of your React app, you can achieve load balancing and distribute the incoming traffic across multiple instances managed by PM2. This setup helps improve performance, scalability, and fault tolerance of your application by utilizing the resources efficiently and handling high traffic loads effectively.
'DevOps' 카테고리의 다른 글
Nginx와 nginx.conf 파일 설정 (0) | 2023.06.18 |
---|---|
Docker & Kubernetes (0) | 2023.01.29 |
AWS EC2 + Docker + AWS ECS를 통한 orchestration (1) | 2023.01.23 |
CI(Continuous Integration)/CD(Continuous Delivery) (0) | 2023.01.11 |
Infra 인프라 (0) | 2022.11.19 |